티스토리 뷰
728x90
반응형
내용
- 서버 통신 시에 네트워크가 유실된 경우 사용자들은 무작정 기다릴 수 밖에 없다. 이때 alert 창과 함께 네트워크 연결 실패에 대해 알려주자!
- Moya 의 Plugin 을 커스텀해서 대응해보자.
Moya Plugin 에 대해서 알아보자
Moya/Plugins.md at master · Moya/Moya
Moya plugin 은 다음의 메서드를 호출해서 request 와 response 를 수정하거나 side-effect 에 대해서 수행할 수 있습니다.
- (
prepare
) after Moya has resolved theTargetType
to aURLRequest
. This is an opportunity to modify the request before it is sent (e.g. add headers). - (
willSend
) before a request is about to be sent. This is an opportunity to inspect the request and perform any side-effects (e.g. logging). - (
didReceive
) after a response has been received. This is an opportunity to inspect the response and perform side-effects. - (
process
) beforecompletion
is called with theResult
. This is an opportunity to make any modifications to theResult
of therequest
.
기존 코드
- Custom Moya Plugin
import Foundation
import Moya
// 🔥 custom Moya Plugin
final class MoyaLoggerPlugin: PluginType {
func willSend(_ request: RequestType, target: TargetType) {
// ...
}
func didReceive(_ result: Result<Response, MoyaError>, target: TargetType) {
switch result {
case let .success(response):
onSuceed(response)
// 🔥 응답을 받는데 실패했다면 네트워크 연결 실패로 alert 창을 띄어보자.
case let .failure(error):
onFail(error)
}
}
func onSuceed(_ response: Response) {
// ...
}
func onFail(_ error: MoyaError) {
var log = "------------------- 네트워크 오류"
log.append("(에러코드: \(error.errorCode)) -------------------\n")
log.append("3️⃣ \(error.failureReason ?? error.errorDescription ?? "unknown error")\n")
log.append("------------------- END HTTP -------------------")
print(log)
}
}
- API
import Foundation
import Moya
public class NoticeAPI {
// 🔥 싱글톤 패턴을 사용.
static let shared = NoticeAPI()
// ✅ Moya plugin 적용.
var noticeProvider = MoyaProvider<NoticeService>(plugins: [MoyaLoggerPlugin()])
// ✅ 객체화할 수 없게 만들어서 싱글톤 패턴으로만 사용하도록 접근 제어자 설정.
private init() { }
func newNoticeFetch(completion: @escaping(NetworkResult<Any>) -> Void) {
noticeProvider.request(.newNoticeFetch) { result in
switch result {
case .success(let response):
// ...
case .failure(let err):
// ...
}
}
}
}
- view controller
private func newNoticeFetchWithAPI(completion: @escaping () -> Void) {
// 🔥 싱글톤 패턴 적용.
NoticeAPI.shared.newNoticeFetch { response in
// ...
}
}
해결
- custom Moya Plugin
import Foundation
import UIKit
import Moya
// 🔥 custom Moya Plugin
final class MoyaLoggerPlugin: PluginType {
// 🔥 alert 창을 띄워줄 view controller 객체를 이니셜라이저를 통해서 초기화.
private let viewController: UIViewController
init(viewController: UIViewController) {
self.viewController = viewController
}
func willSend(_ request: RequestType, target: TargetType) {
// ...
}
func didReceive(_ result: Result<Response, MoyaError>, target: TargetType) {
switch result {
case let .success(response):
onSucceed(response)
// 🔥 응답을 받는데 실패했다면 네트워크 연결 실패로 alert 창을 띄어보자.
case let .failure(error):
onFail(error)
}
}
func onSucceed(_ response: Response) {
// ...
}
func onFail(_ error: MoyaError) {
var log = "------------------- 네트워크 오류"
log.append("(에러코드: \(error.errorCode)) -------------------\n")
log.append("3️⃣ \(error.failureReason ?? error.errorDescription ?? "unknown error")\n")
log.append("------------------- END HTTP -------------------")
print(log)
// 🔥 present alert view controller.
let alertViewController = UIAlertController(title: "네트워크 연결 실패", message: "네트워크 환경을 한번 더 확인해주세요.", preferredStyle: .alert)
alertViewController.addAction(UIAlertAction(title: "확인", style: .default, handler: nil))
viewController.present(alertViewController, animated: true)
}
}
- API
public class NoticeAPI {
// 🔥 이니셜라이저로 전달 받은 view controller 객체를 가지고 MoyaLoggerPlugin 을 초기화.
var noticeProvider: MoyaProvider<NoticeService>
// 🔥 싱글톤 패턴에서 변경.
public init(viewController: UIViewController) {
noticeProvider = MoyaProvider<NoticeService>(plugins: [MoyaLoggerPlugin(viewController: viewController)])
}
func newNoticeFetch(completion: @escaping(NetworkResult<Any>) -> Void) {
noticeProvider.request(.newNoticeFetch) { result in
switch result {
case .success(let response):
// ...
case .failure(let err):
// ...
}
}
}
}
- view controller
private func newNoticeFetchWithAPI(completion: @escaping () -> Void) {
// 🔥 alert 창을 띄울 view controller 객체를 파라미터로 전달.
NoticeAPI(viewController: self).newNoticeFetch { response in
// ...
}
}
구현
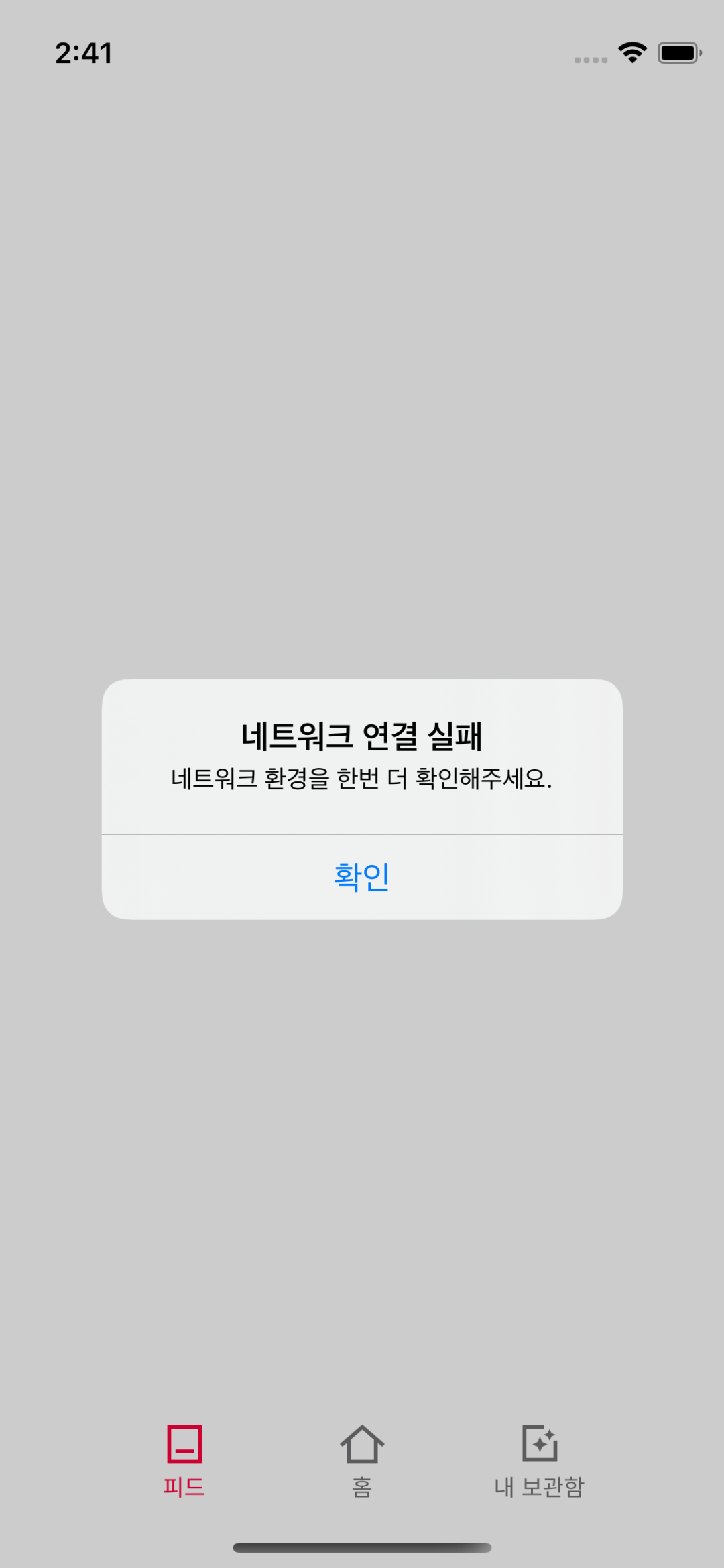
출처:
728x90
반응형
'iOS > Open Library' 카테고리의 다른 글
iOS) RIBs 튜토리얼 1 (0) | 2022.06.02 |
---|---|
iOS) RoundCode 오픈소스 라이브러리를 사용해보자 (0) | 2022.05.27 |
iOS) Moya 에서 Plugin 으로 Token 갱신하기 (0) | 2022.04.11 |
iOS) Moya 에서 DELETE, PATCH 통신 사용해보기 (0) | 2021.10.04 |
iOS) Alamofire 해체쇼 (0) | 2021.09.19 |
댓글
TAG
- async/await
- rxswift
- Protocol
- MVVM
- MOYA
- Firebase
- IOS
- watchOS
- YPImagePicker
- APNS
- CloneCoding
- configurable widget
- 2022 KAKAO TECH INTERNSHIP
- SwiftUI
- urlsession
- RxCocoa
- UserDefaults
- Widget
- containerBackground
- Algorithm
- WidgetKit
- Notification
- Objective-C
- projectsetting
- WWDC
- github
- WWDC22
- 서버통신
- OpenSourceLibrary
- Swift
최근에 올라온 글
최근에 달린 댓글
글 보관함
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | |||||
3 | 4 | 5 | 6 | 7 | 8 | 9 |
10 | 11 | 12 | 13 | 14 | 15 | 16 |
17 | 18 | 19 | 20 | 21 | 22 | 23 |
24 | 25 | 26 | 27 | 28 | 29 | 30 |
링크
- Total
- Today
- Yesterday